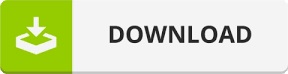

Install it according to the instructions on their website or use SDKMAN or Homebrew. Maven: You need to install Maven to bootstrap the project.
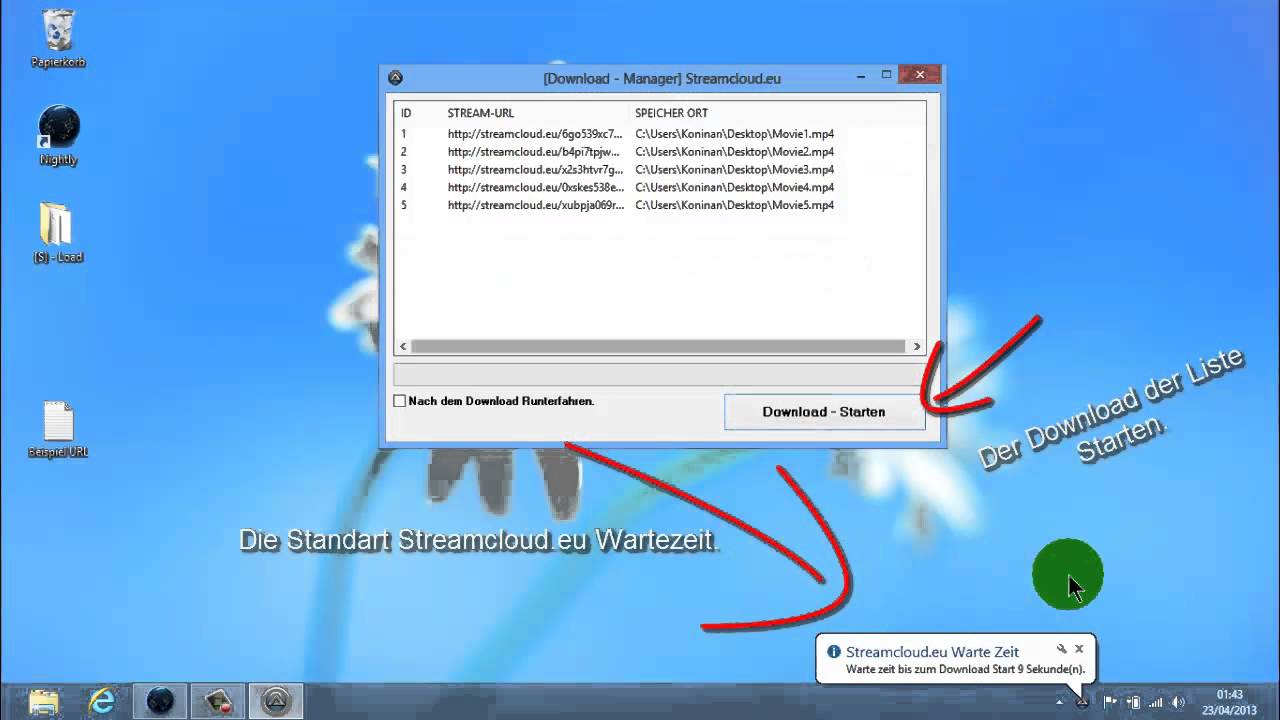
Alternatively, SDKMAN is another great option for installing and managing Java versions. You can find instructions on the OpenJDK website. To do this tutorial, you’ll need a few tools: Set Up a Spring Cloud Stream Development Environment Functional programming is not necessarily reactive, but reactive programming tools almost always use functional programming paradigms. They sometimes return a new stream and sometimes return a reduced result. In reactive programming, you define potentially complex transformations and mappings that you apply to the stream. Reactive programming is a set of tools and techniques that have evolved to treat data as a continuous stream of data, like water flowing through a pipe, rather than viewing them as discrete events to handle individually. Reactive programming involves modeling data and events as observable data streams and implementing data processing routines to react to the changes in those streams. Reactive programming is a programming paradigm that promotes an asynchronous, non-blocking, event-driven approach to data processing. It natively supports reactive programming, which Spring describes as: It uses functional programming techniques to build web services. Spring WebFlux is Spring’s functional web framework that is equivalent to object-oriented Spring MVC. This decouples data and logic in a way that has benefits for applications, such as stream processing, and allows for powerful chaining and composition of functions.
STREAMCLOUD TO CODE
In functional programming, functions, not object instances and classes, are the main organizational unit of code execution. However, you can use Apache Kafka simply by replacing the docker-compose.yml file and by changing the Spring Cloud Stream binding dependency.įunctional programming is a huge departure from the object-oriented model that dominated programming (especially Java) until the last decade. In this tutorial, you will use RabbitMQ in a Docker container. A processor subscribes to a topic and republishes the transformed data to a new topic. Publishers push messages to the topic, and the platform sends the message to all subscribed consumers. A consumer subscribes to a topic (generally just identified by a text name). The simple messaging strategy you’re going to use here is called pub-sub, or publish and subscribe. It excels in stream processing and allows for message storage and replay. Apache Kafka uses a hybrid model (combining aspects of pub-sub and message queuing) that allows you to scale and distribute processing. Very, very briefly: RabbitMQ is a great general-purpose message broker that pushes data from the messaging service to the consumers. They both easily support the simple publish-subscribe (pub-sub) strategy that we will be using in this tutorial however, they are divergent technologies with different strengths and weaknesses. They distribute a stream of messages from producers to consumers. RabbitMQ and Apache Kafka are messaging applications. It’s built on top of Spring Boot, works well with Spring MVC or Spring WebFlux, and can be used to create highly scalable messaging and stream processing applications. It works in distributed microservices that respond to streams of incoming data (data being the “events” in “event-driven”). Spring describes Spring Cloud Stream as “a framework for building highly scalable event-driven microservices connected with shared messaging systems.” This means that the Spring team created Spring Cloud Stream to work with messaging services like RabbitMQ or Apache Kafka. Otherwise, I’m going to take a few paragraphs to introduce the technologies.
STREAMCLOUD TO FREE
If all of that technical jargon made sense to you, feel free to skip to the requirements section. Initially, the messages will be simple types- strings and integers-but you’ll also see how easy Spring Cloud Stream makes mapping POJOs (Plain Old Java Objects) to messages using JSON mapping. The app will use two topics to publish a stream of integers, process the integers to calculate a running total, and consume the processed data. You’ll create an application that contains a publisher, a processor, and a consumer. You’re going to do this using functional, reactive code by utilizing Spring’s WebFlux and by taking advantage of Spring Cloud Stream’s functional binding model.
STREAMCLOUD TO HOW TO
In this tutorial, you’ll learn how to create a Spring Cloud Stream application that interacts with a messaging service, such as RabbitMQ or Apache Kafka.
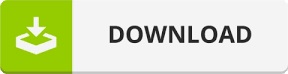